Writing while and for Loops
00:00 In the former lessons, you learned that functions can bundle together code for you, that you can execute with function calls. As you write more and more complex programs, you may find that you need to repeatedly use the same few lines of code.
00:14 You might be tempted to copy and paste similar code to other parts of your program and modify it as needed. But this is usually a bad idea. If you find a mistake in code that’s been copied and pasted all over the place, then you’ll have to apply the fix everywhere the code was copied.
00:31 That’s a lot of work. So yeah, with functions, you can reuse code, but let’s say that you want to shout out loud the word ice cream three times.
00:42 Then even though you’ve got a function, you’d repeat yourself. But worry not! As usual, Python’s got your back.
00:51
A loop is a block of code that repeatedly executes either a specific number of times or until some condition is met. Python has two kinds of loops: while
loops and for
loops. Let’s start with while
loops.
01:04
while
loops repeat a section of code while some condition is true. Let me show you.
01:10
Let’s start by assigning the integer 1
to a variable, n
. Then you create a while
loop by typing the keyword while
followed by a test condition. In this case, n < 5
.
01:28
You close the while
statement with a colon in the end. So this line is called a while
statement. When Python executes a while
loop, it evaluates the test condition and determines if it’s true or false. If the test condition of the while
statement is true, then Python executes the code in the loop body.
01:53 If I’m pressing Enter, I’m in the loop body. The code that will be in the loop body will be run and Python will go on and check the test condition again.
02:06
Let’s first print n
, and in the next line, let’s increase n
by 1
. So the print()
function call and increasing n
is part of the loop body.
02:22 When you press Enter twice, Python executes the loop,
02:27
and as you can see, Python outputs 1
, 2
, 3
, and 4
and then gives you back the prompt. There are five steps taking place.
02:37
First, the value of n
is 1
, so the test condition holds true: 1
is smaller than 5
. So Python steps into the loop body, prints 1
, and increments n
to 2
.
02:51
Then the same thing happens when n
is 2
, 3
, and 4
, until n
has the value 5
.
02:58
When n
is 5
, the test condition is false: 5
is not smaller than 5
. So Python stops the loop, and you can work with the prompt again.
03:08
Let’s verify that n
really has the value 5
. Correct, n
is 5
. Let’s change n
back to 1
because I want to show you something else.
03:20
Recreate the while
loop from before: while n < 5:
… then the loop body, print(n)
. But this time, I don’t increment n
by 1
.
03:37
Do you have an idea what will happen? When n
is not increased, the test condition will always be true. When n
stays 1
, it will always be smaller than 5
.
03:49
If you execute the loop now, you create a so-called infinite loop. That’s a loop that never terminates. The loop body will keep repeating forever, printing the value 1
that n
has over and over again.
04:04
You know what? Let’s do it. Let’s try it out. Ready, go. Enter. And there you go. Now Python prints 1
over and over again. You can see on the right side that the scroll bar gets smaller and smaller.
04:21 When this happened the first time to me, it was a bit scary, and I closed the window. I know, not my proudest moment, because there is other way of terminating this process.
04:33 If something like this happens to you, then you can press Control+C to trigger a keyboard interrupt. It’s the same command on Windows or Unix systems or the mac. It’s always Control+C.
04:51
And with Control+C, Python stops running the program and raises the KeyboardInterrupt
error. And this shortcut works anytime Python is running. As you can see, if I repeatedly press Control+C, then this KeyboardInterrupt
is triggered.
05:11
So yeah, this shortcut is quite handy. Still, you should be careful when you create while
loops and always make sure that you have some kind of exit plan so your loop doesn’t run forever.
05:26
And you will see later that another kind of loop might be a better idea here and there. But before we move to this kind of loop, let me show you a typical example for a while
loop in practice.
05:41
First, the user is prompted to enter a positive number. The test condition determines whether num
is less than or equal to 0
. If num
is positive, then the test condition fails.
05:54
The body of the loop is skipped, and the program ends. Otherwise, if num
is 0
or negative, then the body of the loop executes. The program notifies the user that their input was incorrect and prompts them again to enter a positive number over and over again until the condition fails, and the body of the loop is skipped, and the program ends.
06:21
while
loops are perfect for repeating a section of code while some condition is met. They aren’t well suited, however, for repeating a section of code a specific number of times. That’s where for
loops come into play.
06:35
Let’s look at a for
loop example. Just like the while
loop, the for
loop has two main parts: the for
statement and the loop body. Let’s create a loop that print each letter of the string "donuts"
one at a time. I’ll first type it down, and then we’ll look at it in detail.
06:55
The for
loop starts with the for
keyword, and then I say, letter in
the string "Donut":
Enter print(letter)
.
07:12
And when I hit Enter twice, Python will execute the code and print D
o
n
u
t
.
07:21
Cool. Okay, let’s have a look at it. The for
statement begins with the for
keyword, followed by a membership expression and ending with a colon in the end.
07:35
The loop body contains the code to be executed at each step of the loop. Each line is indented with four spaces. In this example, the for
statement is for letter in "Donut":
.
07:50
The membership expression is letter in "Donut"
. At each step of the loop, the variable letter
is assigned to the next letter in the string "Donut"
.
08:00
And then the value of letter
is printed. The loop runs once for each character in the string "Donut"
. And I think that was the last time I said donut.
08:11
So the loop body executes five times. To see why for
loops are better for looping over collections of items, let’s compare this for
loop with a while
loop.
08:24
I might be biased because of the word donut, but I like the for
loop quite a lot. Let’s compare it to the while
loop, and then you can create your own opinion about it.
08:37
On the left, you can see the for
loop example from our IDLE session again. At each step of the loop, the variable letter
is assigned the next letter in the string "Donut"
, and then the value of the letter
is printed.
08:51
The loop runs once over each character in the string "Donut"
, so the loop executes five times. Let’s look at the right side. To achieve the same thing like in the for
loop, you must create a variable to store the index of the next character in the string.
09:08
At each step of the loop, you print out the character at the current index and then increment the index. The loop will stop once the value of the index
variable is equal to the length of the string. That’s significantly more complex than the for
loop version.
09:26
Let’s have a look at the first while
loop example from before and see how we can rewrite it with a for
loop.
09:35
When you want to loop over a range of numbers, Python has a handy built-in function called range()
. The range()
function produces a range of numbers.
09:46
range()
loops from 0
to the number you provide as an argument. You can also give range a starting point. For example, range(1, 5)
is the range of numbers 1
, 2
, 3
, and 4
. The first argument is the starting point, and the second argument is the endpoint, which is not included in the range.
10:09
When Python runs the loop on the left side, you print the numbers 1
, 2
, 3
, and 4
. Let’s have a look at the while
loop version on the right. First, you assign the integer 1
to a variable, n
. When Python executes a while
loop, it evaluates the test condition and determines if it is true or false.
10:31
If the test condition is true, then Python executes the code in the loop body and returns to check the test condition again. Here you check whether the value of n
is less than 5
.
10:43
If n
is less than 5
, then the body of the loop is executed: you print n
and increase the value of n
by 1
.
10:52
So the output is exactly the same as the output of the for
loop that you can see on the left. You print 1
, 2
, 3
, and 4
.
11:01
But again, the code of the while
loop is way more complicated.
11:07
So yeah, most of the time, a for
loop is more concise and easier to read than an equivalent while
loop. That’s why you’ll probably encounter and use for
loops much more often than while
loops. Before we wrap this lesson up, let me show you another cool thing.
11:26
As long as you indent the code correctly, you can even put loops inside of other loops. So for example, for n in range(1, 4):
Enter … So now I’m indented with four spaces, and I create another for
loop, for j in range(
… again, 1, 4):
Enter.
11:56 And now you see I’m indented eight spaces.
12:00
print(
… starting an f-string …
12:17
closing the string, closing the parentheses, and hitting Enter once, twice, and now you can see a nice long printed output saying n = 1 and j = 1
, n = 1 and j = 2
, and so on until n = 3 and j = 3
.
12:39
So when Python executes the first loop, it assigns the value 1
to the variable n
. Then it executes the second for
loop and assigns the value 1
to j
. The first result is n = 1
and j = 1
.
12:57
After executing print()
, Python returns to the inner for
loop and assigns 2
to j
and prints n = 1
and j = 2
. Python doesn’t return to the outer for
loop, because the inner for
loop, which is inside the body of the outer for
loop, isn’t done executing.
13:18
So next, Python assigns 3
to j
and prints n = 1
and j = 3
. At this point, the inner for
loop is done executing, so control returns to the outer for
loop. Python assigns the value 2
to variable n
, and then the inner for
loop executes a second time.
13:41
This process repeats a third time when n
is assigned the value 3
. A loop inside another loop is called a nested loop, and it comes up more often than you might expect.
13:53
You can nest while
loops inside for
loops and vice versa. You can even nest loops more than two levels deep. But you heard me saying this a few times during this course: if you nest your loops, then you have to take care of the indentation of your code.
14:13
All right, now you know a thing or two about while
loops and for
loops. But didn’t somebody say ice cream at the beginning of this lesson? Yeah, you’re right. So there is one last thing that we need to do.
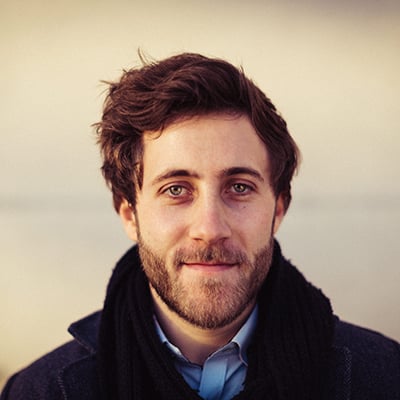
Philipp Acsany RP Team on Aug. 3, 2023
Hi Jesús! When you close the window you terminate the process. That’s the good news. However, I’m curious about why ctrl + c didn’t work for you. What operating system are you on?
(Sometimes it also works to hit ctrl + c twice to really terminate the processes. If you’re still up for it, you may try this out, too.)
Maya Loco on May 1, 2024
Really funny with the endless loop! Too bad IDLE wont agree with that. It stops responding so I had to end the application. ICE CREAM! FYI. Running IDLE 3.12.1 on Windows.
Become a Member to join the conversation.
Jesús Pineda on Aug. 1, 2023
I did the infinity loop test!! and the command ctrl + c.,ctr + z, ctrl + x, It never worked,a couple of times. I resorted to closing the window and the program, I hope it hasn’t been running in the background or something like that!.Any other recommendation, when that happens, what to do if the command does not respond?